You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
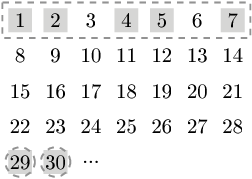
Write a function:
int solution(int A[], int N);
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
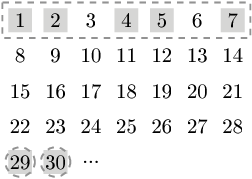
Write a function:
int solution(vector<int> &A);
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
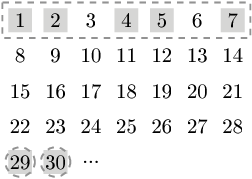
Write a function:
class Solution { public int solution(int[] A); }
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
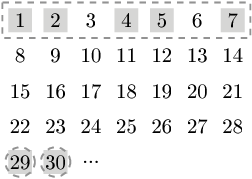
Write a function:
int solution(List<int> A);
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
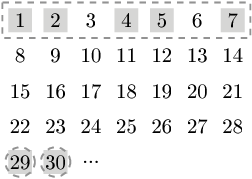
Write a function:
func Solution(A []int) int
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
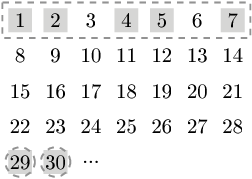
Write a function:
class Solution { public int solution(int[] A); }
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
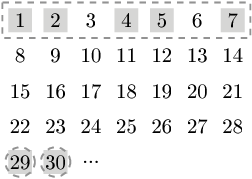
Write a function:
class Solution { public int solution(int[] A); }
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
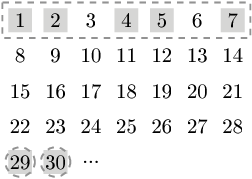
Write a function:
function solution(A);
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
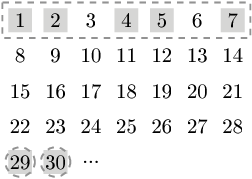
Write a function:
fun solution(A: IntArray): Int
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
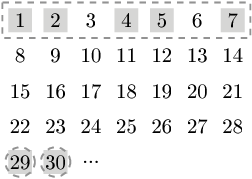
Write a function:
function solution(A)
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Note: All arrays in this task are zero-indexed, unlike the common Lua convention. You can use #A to get the length of the array A.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
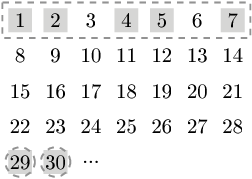
Write a function:
int solution(NSMutableArray *A);
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
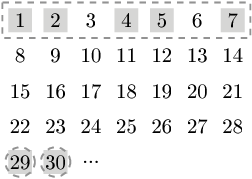
Write a function:
function solution(A: array of longint; N: longint): longint;
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
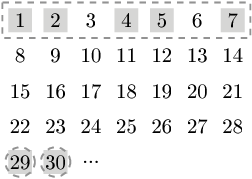
Write a function:
function solution($A);
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
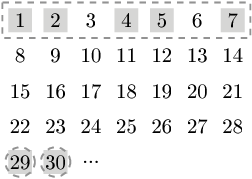
Write a function:
sub solution { my (@A) = @_; ... }
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
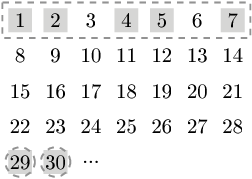
Write a function:
def solution(A)
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
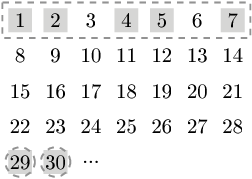
Write a function:
def solution(a)
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
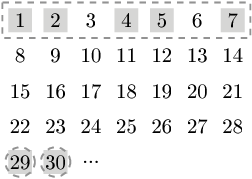
Write a function:
object Solution { def solution(a: Array[Int]): Int }
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
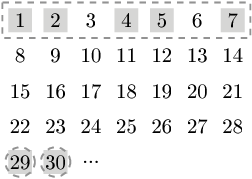
Write a function:
public func solution(_ A : inout [Int]) -> Int
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
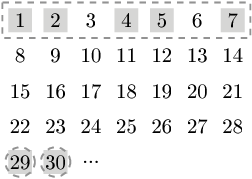
Write a function:
function solution(A: number[]): number;
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
You want to buy public transport tickets for some upcoming days. You know exactly the days on which you will be traveling. There are three types of ticket:
- 1-day ticket, costs 2, valid for one day;
- 7-day ticket, costs 7, valid for seven consecutive days (e.g. if the first valid day is X, then the last valid day is X+6);
- 30-day ticket, costs 25, valid for thirty consecutive days.
You want to pay as little as possible having valid tickets on your travelling days.
You are given a sorted (in increasing order) array A of days when you will be traveling. For example, given:
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
you can buy one 7-day ticket and two 1-day tickets. The two 1-day tickets should be used on days 29 and 30. The 7-day ticket should be used on the first seven days. The total cost is 11 and there is no possible way of paying less.
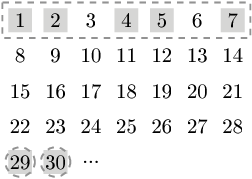
Write a function:
Private Function solution(A As Integer()) As Integer
that, given an array A consisting of N integers that specifies days on which you will be traveling, returns the minimum amount of money that you have to spend on tickets.
For example, given the above data, the function should return 11, as explained above.
Write an efficient algorithm for the following assumptions:
- M is an integer within the range [1..100,000];
- each element of array A is an integer within the range [1..M];
- N is an integer within the range [1..M];
- array A is sorted in increasing order;
- the elements of A are all distinct.
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
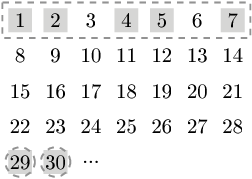
下記の関数を実装してください。
int solution(int A[], int N);
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
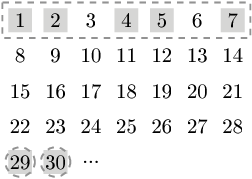
下記の関数を実装してください。
int solution(vector<int> &A);
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
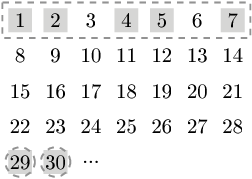
下記の関数を実装してください。
class Solution { public int solution(int[] A); }
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
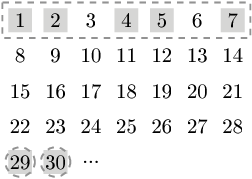
下記の関数を実装してください。
int solution(List<int> A);
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
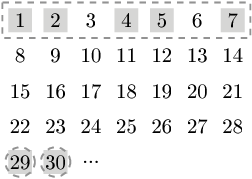
下記の関数を実装してください。
func Solution(A []int) int
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
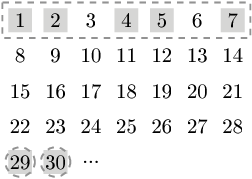
下記の関数を実装してください。
class Solution { public int solution(int[] A); }
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
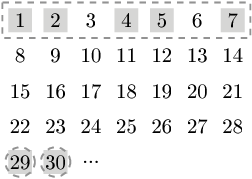
下記の関数を実装してください。
class Solution { public int solution(int[] A); }
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
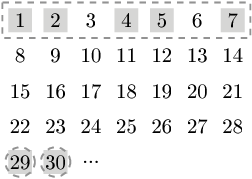
下記の関数を実装してください。
function solution(A);
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
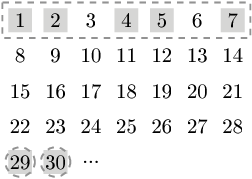
下記の関数を実装してください。
fun solution(A: IntArray): Int
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
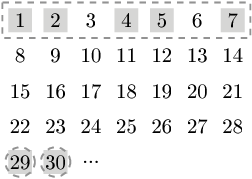
下記の関数を実装してください。
function solution(A)
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
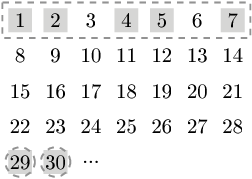
下記の関数を実装してください。
int solution(NSMutableArray *A);
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
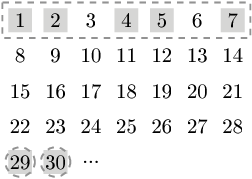
下記の関数を実装してください。
function solution(A: array of longint; N: longint): longint;
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
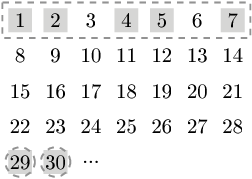
下記の関数を実装してください。
function solution($A);
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
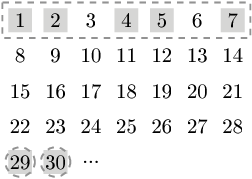
下記の関数を実装してください。
sub solution { my (@A) = @_; ... }
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
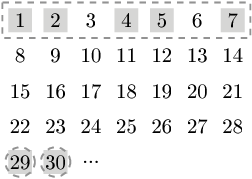
下記の関数を実装してください。
def solution(A)
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
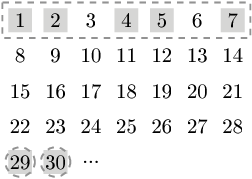
下記の関数を実装してください。
def solution(a)
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
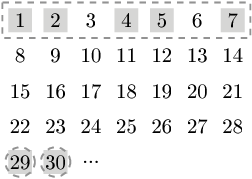
下記の関数を実装してください。
object Solution { def solution(a: Array[Int]): Int }
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
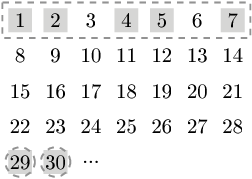
下記の関数を実装してください。
public func solution(_ A : inout [Int]) -> Int
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
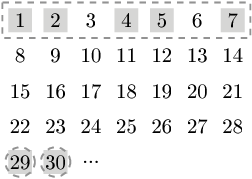
下記の関数を実装してください。
function solution(A: number[]): number;
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.
来る旅行のために、公共交通機関のチケットを買いたいとします。旅行日程はもう確定しています。 3種類のチケットがあるとします。
- 1種類目は、 ワンデーチケット。値段は2で、1日のみ有効です。
- 2種類目は、7日間チケット。値段は7で、連続した7日間有効 です。 (たとえば、有効期間の初日がXdayとすると、有効期間の最終日は、X+6となります)
- 3種類目は、月間チケット(30日間)。値段は25で、連続した30日間有効 です。
あなたは旅行で使うチケットの負担を出来る限り最小限に抑えたいとします。 ここに、あなたの旅行日程数が、昇順に収められた、配列Aが与えられるとします。たとえば以下のように。
A[0] = 1
A[1] = 2
A[2] = 4
A[3] = 5
A[4] = 7
A[5] = 29
A[6] = 30
この場合、あなたは、7日間チケットとワンデーチケットを2つ買うことができます。 2つのワンデーチケットは、29日と30日に利用され、7日間チケットは、初日から7日間で使われることが想定されます。 この場合チケットにかかる合計の値段は11となり、これ以上安くする方法はありません。
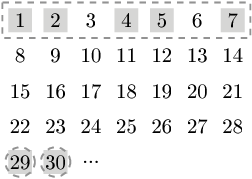
下記の関数を実装してください。
Private Function solution(A As Integer()) As Integer
この関数は、N個の整数を持つゼロからはじまる配列A(この配列は旅行日数を表します)が与えられた時、あなたがチケットを買うために支払わなければならない最小金額を返します。 例えば前述の データをいれる場合、11が返却されます。※前述の通り
Mは配列の最も大きい要素を表すとします。 つまり、旅行予定日の最大数です。
前提条件:
- M は [1..100,000] の範囲の整数とする。
- 配列 A の各要素は [1..M] の範囲の 整数 とする。
- N は [1..M] の範囲の整数とする。
- Aの配列は昇順ソートされ
- A の要素は互いに異なる
Copyright 2009–2025 by Codility Limited. All Rights Reserved. Unauthorized copying, publication or disclosure prohibited.